Synfig studio app download. Working on the user interface can take disproportionate amount of time. I usually do the logic work first, and UI last, in the spare time. In the smart mirror project the UI is very bare bones: it’s only here to test data bindings. Let’s improve the looks of it.
A quick reference guide to common markup extensions used in Xamarin Forms XAML. Xamarin’s intellisense is not as powerful as WPF’s, nor are the markup extensions of XAML the same between WPF or Xamarin Forms and if you come from a web background, XAML may seem rather confusing at first. WPF XAML Data Binding Cheat Sheet One of the best things about WPF is the advanced data binding support, the Binding class that is used to create data bindings has many interesting and useful options. And I never remember the more advanced options when I’m writing XAML. Within this page is a table containing a complete list of all Segoe UI Symbol characters. I used this a lot and found it very useful, but one thing it seemed to be missing was details about the names of the buttons within the Common/StandardStyles.xaml file. Without these names, it was hard to search through the symbols looking for one that. Contribute to tatsuyafujisaki/wpf-cheat-sheet development by creating an account on GitHub.
Table of contents:
I wrote this blog post in preparation and after shooting a video about XAML. You can watch me go through these steps:
The biggest bang for the buck for the smart mirror project is to request a dark theme. In App.xaml
:
change this into
Drastically changes the appearance of the app:
It’s a good practice to keep related code together and unrelated code separately. We will leave the viewmodels in the main App.xaml
and move style-related code to its own file.
Create a new Resource Dictionary (right click on a project, Add New Item > XAML > Resource Dictionary). If you call it MyApp.xaml
and put it in Themes
, use the following code in App.xaml
: Add <ResourceDictionary.MergedDictionaries>
where you will specify the path to the new dictionary.
Important notice: If you had some resources stored directly in <Application.Resources>
before, now you need to move all resources into the <ResourceDictionary>
.
Let’s try to theme all instances of TextBlock
. To do this, create a Style
with TargetType
but without the Key
. You can put it either into ResourceDictionary
in App.xaml
or MyApp.xaml

This works for free-standing TextBlocks
, but not for these embedded in a template, e.g. DataTemplate
To style absolutely all instances of TextBlock
, we need to set each one’s Style
property. It’s unfortunate that it requires much more code changes and it’s not as elegant as CSS tag selector. Let’s take advantage of this, though, and create different styles for different TextBlocks
. XAML styles implement inheritance with BasedOn
property:
To use these styles, assign appropriate StaticResource
to element’s Style
. Here we’re using all three styles for various TextBlocks
:
We can also override the resources used by the default theme. Unfortunately, we can’t set the color of TextBlocks (their color is hard-coded rather than provided by another resource).
For the sake of showing an example, we will modify application’s background which is provided by a SolidColorBrush
with Key='ApplicationPageBackgroundThemeBrush'
. All we need to do is create a new resource with the same Key
as resources found in the base theme, and put it in correct hierarchy of resources. Specifically, we put the resource in ResourceDictionary
which
- Is inside
<ResourceDictionary.ThemeDictionaries>
- this tells XAML that we want to override resources that make up one of the three base themes:Light
,Dark
orHighContrast
- Has the key
'Default'
for the dark theme,'Light'
for the light theme, and'HighContrast'
for the high contrast theme.
How did I know to change ApplicationPageBackgroundThemeBrush
?
The base themes are available for your reference in generic.xaml
and themeresources.xaml
in C:Program Files (x86)Windows Kits10DesignTimeCommonConfigurationNeutralUAP10.0.10586.0Generic
(the version number might be different)
Explore these files to find resources that base styles depend on. You can change them to easily theme large chunks of your app.
Think again if you really want to do it. There is no nice solution
I would like the theme of the app to use a red color at night. In Universal Windows Apps there is no DynamicResource
, so changing properties of resources is not as elegant as in regular Win32 app. If you want to look at all failed attempts to get it working, here is my SO question.
The following options don’t work in Universal Windows App:
- Using
DynamicResource
- This type is no longer used, for performance reasons
- Changing a dictionary at runtime
- As demonstrated in Dynamically Skinning Your Windows 8 App
- Using an Effect to change colors
Effects
are also removed fromUIElements
Here’s what you can do:
- Change element’s
Style
- To enumerate all elements, use this code
- Change the element’s theme
- As there are only Light and Dark themes, you don’t have much flexibility.
We will change the theme of the Frame
that contains the entirety of the user interface. For the complete solution, read this SO answer. In brief:
- Create and use
ThemeAwareFrame
that inherits fromFrame
(code is in the SO answer)
- Create your resource in both Light and Dark themes
- Change the
AppTheme
of the instance ofThemeAwareTheme
. In this example, we’re callingTimeOfDayChangedHandler
every second with alternating boolean flag.
Here’s the result:
So far so good. This is the first time I was able to change anything on the screen! Now we need to overwrite the light theme such that it looks like the dark theme. Notice that this will force users of your app to use the dark theme, despite their personalized system settings. This will also force you to stick to one theme, as the other one will be sacrificed for the effect.
- Go to
C:Program Files (x86)Windows Kits10DesignTimeCommonConfigurationNeutralUAP10.0.10586.0Genericthemeresources.xaml
- Copy it into a new dictionary in your solution:
Themes/LightOverride.xaml
- Remove all nodes except for
<ResourceDictionary x:Key='Default'>
(dark theme) - Rename this node to
<ResourceDictionary x:Key='Light'>
(make it the light theme) - Merge this dictionary in App.xaml
Now, we are done. Notice the letters change from light blue to light yellow.
When displaying weather forecast, I don’t want to show “0mm rain” on each sunny day. We can use a converter to hide this text.
Create a class NonZeroValueToVisibilityConverter
in namespace My.App.Converters
, add it to the dictionary in the following way:
And use it in the following way:
This screenshot was taken halfway through UI redesign. The rain and snow quantities are shown only when they’re non-zero.
The XML documentation tags of C# are described very well in the MSDN. However, the article does not explain how the contained phrases and sentences should be written. This article tries to fill this gap by providing rules and some sample phrases.
I recommend using StyleCop because its rules enforce some of the XML documentation recommendations from this article. Also check out the Visual Studio extension GhostDoc which automates and simplifies the writing of XML documentation.
General
All XML documentation phrases should end with a period (.) and no blank:
The
summary
tags should only contain the most important information. For further details use an additionalremarks
tag. To avoid having too much documentation in your source code files, read this article which explains how to “outsource” documentation to an external file.
Classes
Each class should have a
summary
tag describing its responsibility. The summary often starts with “Represents …“ or “Provides …“ but other forms also exist:
Constructors
The documentation of a constructor should be in the form “Initializes a new instance of the <see cref=”TYPENAME”/> class.”</see>:
Properties
The summary of a property should start with “Gets or sets …“ if it is fully accessible, with “Gets …“ when the property is read-only, and with “Sets …“ if it is write-only:
Note: According to the very recommended book Framework Design Guidelines, write-only properties should not be used at all.
A property of type
bool
should start with “Gets or sets a value indicating whether …“:
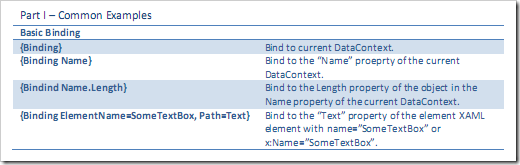
Events
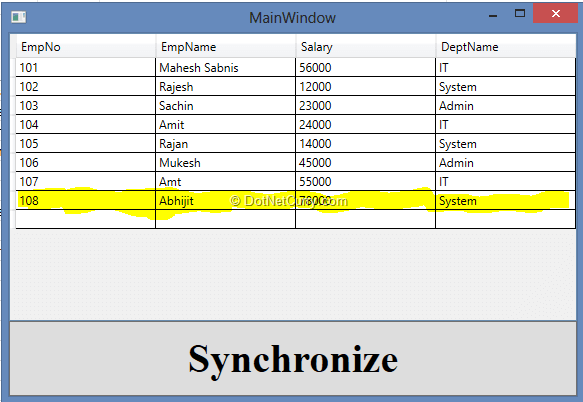
Each event should have a
summary
tag which starts with the words “Occurs when …“:Note: Events should be named with a verb or verb phrase. Use the present and past tenses to express if the delegate is invoked before (e.g.
Closing
) or after (e.g.Closed
) a particular event.
Methods
Like the method name itself, the text in the
summary
tag should start with a verb:
Method parameters
Xaml Cheat Sheet

Each method parameter should have a corresponding
param
tag containing a description of the parameter:If the parameter is an
enum
or of typebool
consider starting the description with “Specifies …“ (enum) or “Specifies whether …“ (bool)Note: According to Clean Code you should not use
bool
method parameters.
Method return value
If the method returns a value, you should add a
returns
tag which contains a description of the returned object:If the returned value is of type
bool
the documentation should be in the form “true if CONDITION; otherwise,false .”:
Exceptions
The exception
exception
tag contains the reason why the exception occurred:Tip: Have a look at Exceptional for ReSharper which greatly helps creating XML documentation for exceptions.
Final words
Xaml Stringformat Integer
The rules in this articles are used in StyleCop and in the .NET Framework, therefore I think using them is best practice. Because it looks more clean to me, I write the opening tag, content and closing tag on a single line. Of course you can write them on multiple lines as proposed by the Visual Studio templates. For the generated XML documentation file it does not matter what style you are using.
Xaml Cheat Sheet
What do you think about the described XML documentation rules? Are some rules missing in this list?
Rico Suter SOFTWARE ENGINEERING EDIT
Best PracticesXML Documentation.NETC#Cheat SheetClean CodeCoding GuidelinesDocumentation
Xaml Cheat Sheet Pdf
Please enable JavaScript to view the comments powered by Disqus.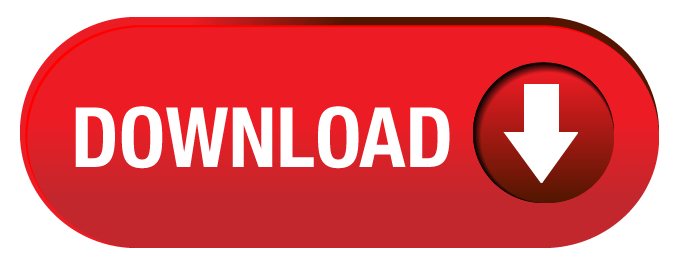